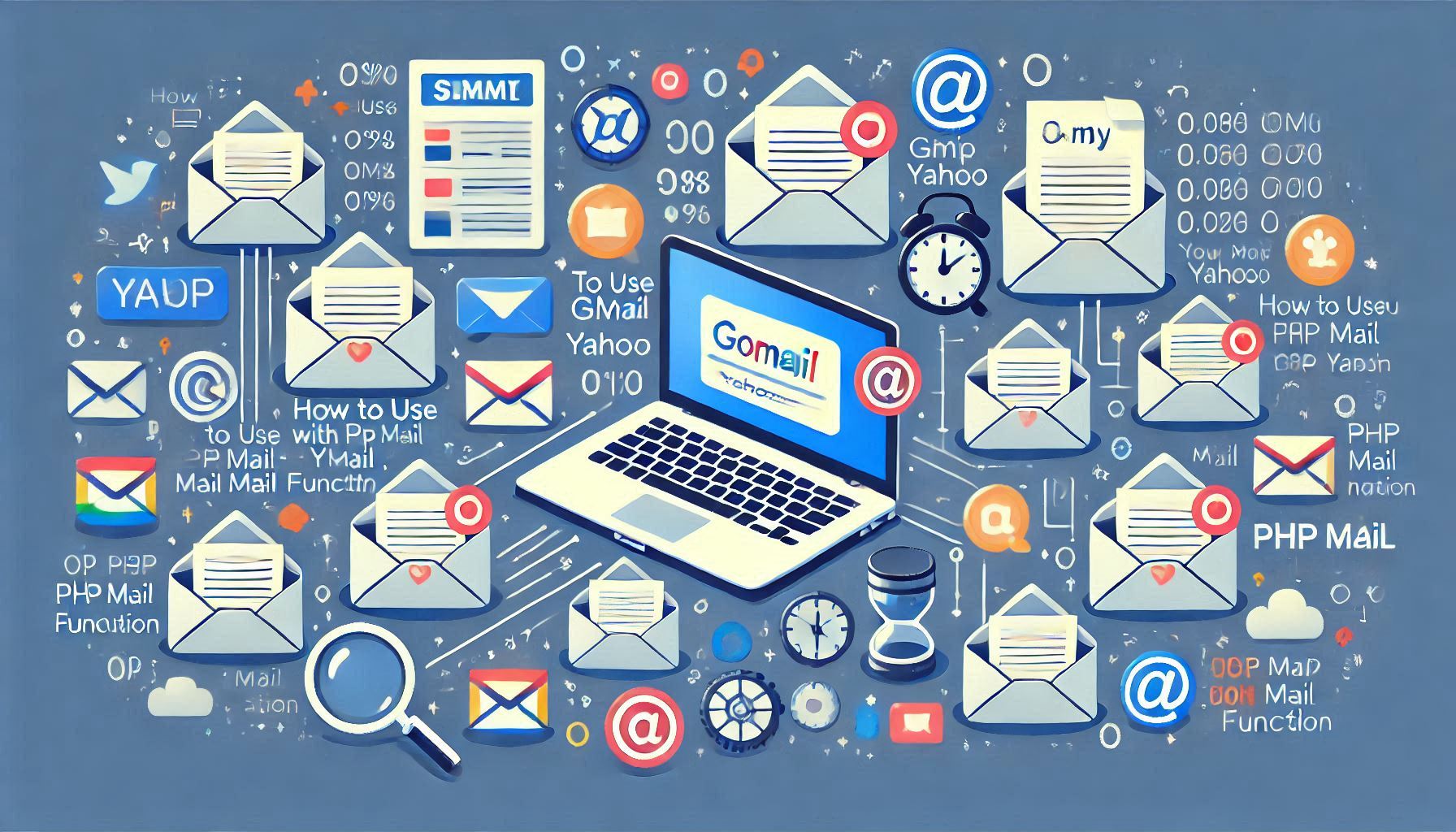
How To Use Gmail or Yahoo with PHP mail() Function
In the world of web development, sending emails programmatically is a common requirement. Whether it’s for sending notifications, alerts, or newsletters, the PHP mail()
function has been a staple for many developers. However, when it comes to using email accounts from providers like Gmail or Yahoo, you need to implement additional steps to ensure your emails are sent reliably and securely. In this blog post, we’ll explore how to leverage Gmail and Yahoo for sending emails using PHP’s mail()
function.
Understanding the PHP mail() Function
The mail()
function in PHP is a simple way to send emails directly from a script. The basic syntax is as follows:
mail(to, subject, message, headers);
- to: Recipient’s email address.
- subject: Subject of the email.
- message: The body of the email.
- headers: Additional headers, such as
From
,Cc
, andBcc
.
However, using the mail()
function in its basic form can lead to issues, especially when sending emails through popular providers like Gmail and Yahoo. Emails may end up in the spam folder or, worse, not be sent at all.
Why Use SMTP Instead?
Though using the mail()
function is straightforward, it doesn’t support SMTP authentication out of the box, which is required by Gmail and Yahoo for sending emails securely. To overcome this limitation, we recommend using libraries like PHPMailer or SwiftMailer, which provide an easy way to send emails via SMTP.
Sending Emails Using Gmail SMTP
Step 1: Enable Less Secure Apps
Before you can use your Gmail account to send emails, you need to allow access to less secure apps. Here’s how:
- Log in to your Gmail account.
- Go to your Google Account settings.
- Navigate to the “Security” section.
- Scroll down to “Less secure app access” and turn it ON.
Note: Google is phasing out less secure app access, so consider using App Passwords or OAuth2 for better security.
Step 2: Install PHPMailer
You can install PHPMailer via Composer. If you haven’t set up Composer, you can download PHPMailer from GitHub.
composer require phpmailer/phpmailer
Step 3: Write the PHP Script
Here’s a sample PHP script that uses PHPMailer to send an email using Gmail:
<?php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'vendor/autoload.php';
$mail = new PHPMailer(true);
try {
//Server settings
$mail->isSMTP();
$mail->Host = 'smtp.gmail.com';
$mail->SMTPAuth = true;
$mail->Username = '[email protected]'; // Your Gmail address
$mail->Password = 'your_password'; // Your Gmail password or App Password
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587;
//Recipients
$mail->setFrom('[email protected]', 'Your Name');
$mail->addAddress('[email protected]', 'Recipient Name');
// Content
$mail->isHTML(true);
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
?>
Step 4: Run Your Script
Save your script on your server and execute it. Ensure that your server is configured to allow outbound connections on port 587.
Sending Emails Using Yahoo SMTP
The process for sending emails via Yahoo’s SMTP is quite similar to Gmail. Here’s how to do it:
Step 1: Enable App Passwords
- Log into your Yahoo account.
- Go to Account Security settings.
- Under “Other App Passwords”, generate a new password for your application.
Step 2: Modify Your Script
Replace the Gmail-specific settings in your PHPMailer script with Yahoo’s SMTP settings:
$mail->Host = 'smtp.mail.yahoo.com';
$mail->Username = '[email protected]';
$mail->Password = 'your_app_password'; // Use the App Password you just generated
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->Port = 587;
Step 3: Run Your Script
Just like with Gmail, ensure your script is executed in a suitable environment that allows outgoing SMTP connections.
Conclusion
Using the PHP mail()
function directly with Gmail or Yahoo is not recommended due to limitations in security and reliability. By using PHPMailer and connecting via SMTP, you can ensure that your emails are sent securely and are less likely to be marked as spam.
At Greenhost.cloud, we believe in empowering developers with the right tools and knowledge. If you have any questions or need further assistance, feel free to reach out to us!
Happy coding, and may your emails always reach their destination!