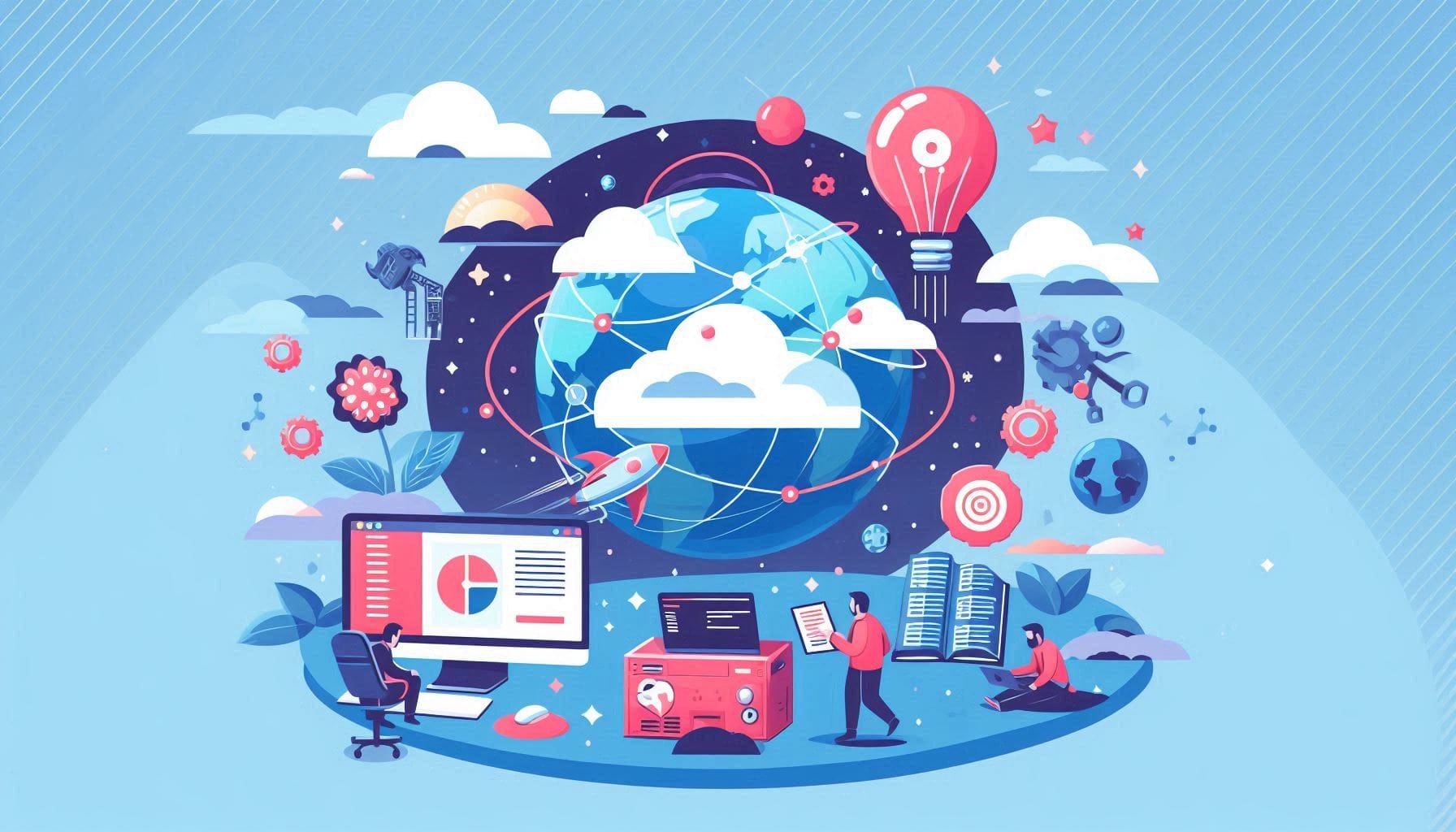
How To Deploy a Local Django App to an Ubuntu 24.04 Server
Deploying a Django app can seem daunting at first, but with the right guidance, you can have your application up and running on an Ubuntu 24.04 server in no time. In this blog post, we will walk you through the necessary steps to successfully deploy your local Django application. Let’s get started!
Prerequisites
Before we dive into the deployment process, ensure you have the following:
- A Local Django Application: Make sure your app is working perfectly on your local machine.
- An Ubuntu 24.04 Server: You can use a cloud provider like Greenhost to set one up.
- SSH Access: You should have SSH access to your server.
- Basic knowledge of the command line: Familiarity with terminal commands will be helpful.
Step 1: Connect to Your Server
Start by connecting to your server via SSH. Open your terminal and run:
ssh username@your_server_ip
Replace username
with your server’s username and your_server_ip
with the actual IP address.
Step 2: Update Your Server
Once logged in, it’s a good practice to update your server’s package index. Run:
sudo apt update
sudo apt upgrade -y
Step 3: Install Dependencies
Django requires a few packages to function correctly. Install the necessary dependencies:
sudo apt install python3-pip python3-dev libpq-dev nginx curl git
- python3-pip: Python package manager.
- python3-dev: Development files for Python.
- libpq-dev: PostgreSQL library for Python.
- nginx: A web server to serve your application.
- curl: Command-line tool for transferring data with URLs.
- git: Version control system to manage your code.
Step 4: Install and Set Up a Virtual Environment
It’s best to use a virtual environment for your Django application. Install the virtualenv
package if you haven’t already:
pip3 install virtualenv
Now, create a directory for your app and set up a virtual environment:
mkdir ~/myproject
cd ~/myproject
virtualenv venv
Activate the virtual environment:
source venv/bin/activate
Step 5: Clone Your Django Application
If your Django app is stored in a Git repository, you can clone it directly onto your server:
git clone https://github.com/yourusername/your-django-app.git .
If you are not using Git, you can upload your files using scp
or any file transfer method you prefer.
Step 6: Install Python Dependencies
Make sure you have a requirements.txt
file in your project directory. Install the required packages:
pip install -r requirements.txt
Step 7: Configure Database Settings
If you are using PostgreSQL (or any other database), make sure to set up your database and configure your Django settings to connect to it. For PostgreSQL, you can create a new database:
sudo -u postgres psql
In the PostgreSQL shell:
CREATE DATABASE mydatabase;
CREATE USER myuser WITH PASSWORD 'mypassword';
ALTER ROLE myuser SET client_encoding TO 'utf8';
ALTER ROLE myuser SET default_transaction_isolation TO 'read committed';
ALTER ROLE myuser SET timezone TO 'UTC';
GRANT ALL PRIVILEGES ON DATABASE mydatabase TO myuser;
\q
Then, update your settings.py
file in your Django project to use the new database settings.
Step 8: Run Migrations
Apply your database migrations:
python manage.py migrate
Step 9: Collect Static Files
Django needs to serve static files (CSS, JavaScript, images, etc.) separately. Run the following command:
python manage.py collectstatic
Step 10: Test the Application
You can run the development server to test your application:
python manage.py runserver 0.0.0.0:8000
Open your browser and navigate to http://your_server_ip:8000
to see if your app is working.
Step 11: Set Up Gunicorn
Now, let’s set up Gunicorn, a WSGI HTTP server for UNIX. Install it using pip:
pip install gunicorn
You can run your Django application with Gunicorn like this:
gunicorn --bind 0.0.0.0:8000 myproject.wsgi:application
Step 12: Configure Nginx
Create a new Nginx configuration file for your Django app:
sudo nano /etc/nginx/sites-available/myproject
Add the following configuration:
server {
listen 80;
server_name your_server_ip;
location = /favicon.ico { access_log off; log_not_found off; }
location /static/ {
root /home/username/myproject;
}
location / {
include proxy_params;
proxy_pass http://unix:/home/username/myproject/myproject.sock;
}
}
Replace your_server_ip
and username
with your actual server details.
Enable the new configuration by creating a symbolic link:
sudo ln -s /etc/nginx/sites-available/myproject /etc/nginx/sites-enabled
Test the Nginx configuration:
sudo nginx -t
If everything is okay, restart Nginx:
sudo systemctl restart nginx
Step 13: Start Gunicorn with Systemd
Create a systemd service file for Gunicorn:
sudo nano /etc/systemd/system/myproject.service
Add the following content:
[Unit]
Description=gunicorn daemon for myproject
After=network.target
[Service]
User=username
Group=www-data
WorkingDirectory=/home/username/myproject
Environment="PATH=/home/username/myproject/venv/bin"
ExecStart=/home/username/myproject/venv/bin/gunicorn --access-logfile - --workers 3 --bind unix:/home/username/myproject/myproject.sock myproject.wsgi:application
[Install]
WantedBy=multi-user.target
Enable and start the Gunicorn service:
sudo systemctl start myproject
sudo systemctl enable myproject
Conclusion
Your Django application is now deployed and accessible via your server’s IP address! You can further secure your application with SSL and domain name configuration, but this guide provides the foundational steps to get your app running on an Ubuntu 24.04 server.