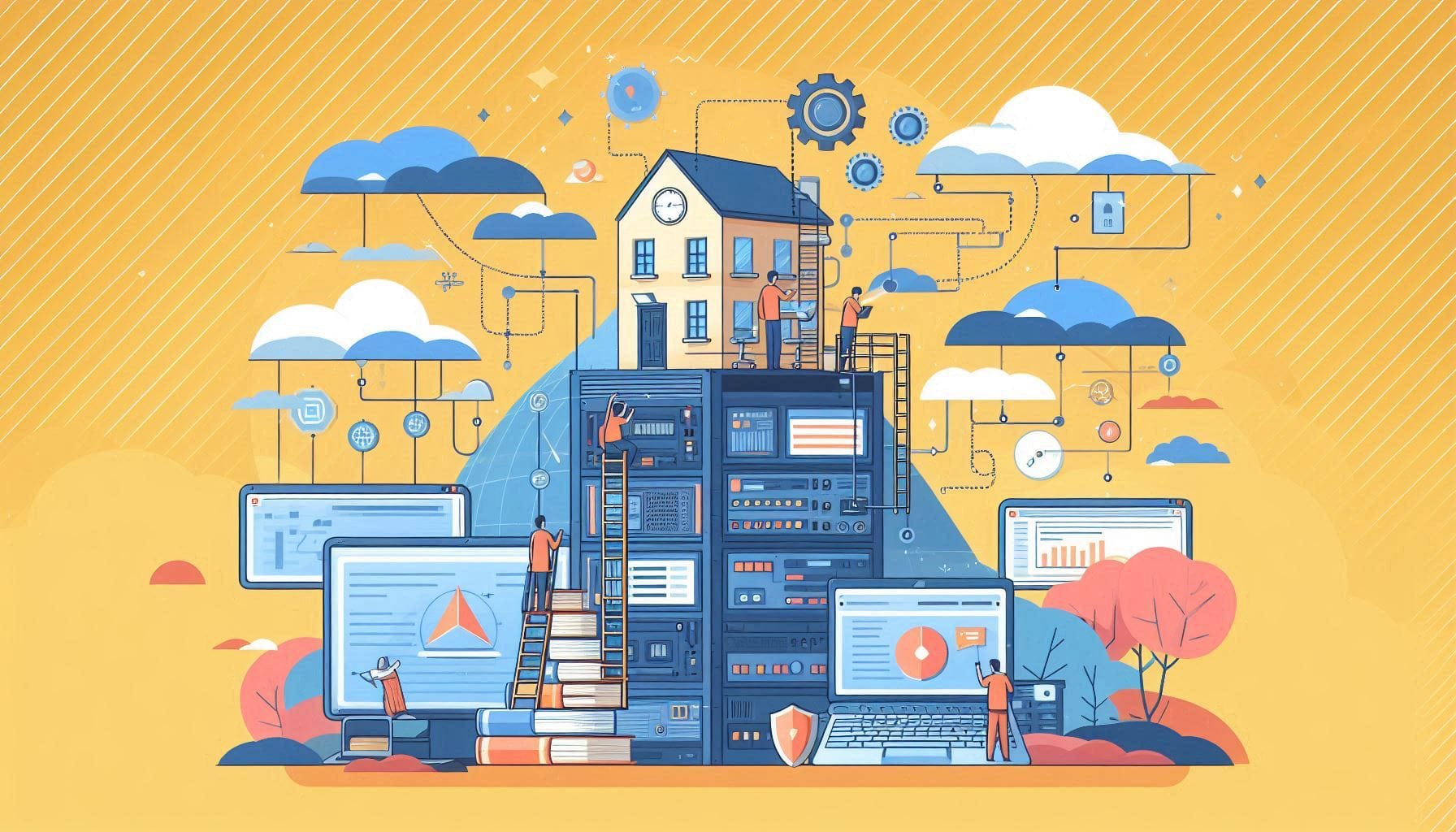
How To Install And Run A Node.js App On CentOS
Welcome to the Greenhost.cloud blog! In today’s post, we’re going to walk you through the steps on how to install and run a Node.js application on a CentOS server. Node.js is a powerful platform that allows you to build scalable network applications in JavaScript. Whether you’re a seasoned developer or just getting started, this guide will help you set up your environment correctly so you can hit the ground running.
Step 1: Update Your System
First things first, you’ll want to ensure your CentOS system is up to date. Open your terminal and log in to your CentOS server. Once you’re in, run the following command:
sudo yum update -y
This command updates all the packages on your system, ensuring that you have the latest security updates and improvements.
Step 2: Install Node.js and npm
Node.js can be installed in different ways, but one of the most straightforward methods is to use the NodeSource repository. Follow these steps to install Node.js:
- Add the NodeSource repository: Before you can install Node.js, you need to add the NodeSource repository. The following command adds the repository for Node.js version 14.x, but you can replace
14
with your desired version number:
curl -sL https://rpm.nodesource.com/setup_14.x | sudo bash -
- Install Node.js: After adding the repository, you can install Node.js and npm (Node Package Manager) with:
sudo yum install -y nodejs
- Verify the Installation: Once the installation completes, confirm Node.js and npm were installed correctly by checking their versions:
node -v
npm -v
You should see the version numbers printed in your terminal.
Step 3: Create a Simple Node.js Application
Now that you’ve installed Node.js, let’s create a simple application to test that everything is working fine.
- Create a new directory for your application:
mkdir my-node-app
cd my-node-app
- Initialize a new Node.js project: This step will generate a
package.json
file, which will keep track of your project dependencies.
npm init -y
- Create your main application file: Create a file named
app.js
in your project directory:
touch app.js
Open app.js
with your preferred text editor and add the following basic server code:
const http = require('http');
const PORT = 3000;
const requestHandler = (req, res) => {
res.end('Hello, World!');
};
const server = http.createServer(requestHandler);
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Step 4: Run Your Node.js Application
Now, you’re ready to run your Node.js application! In your terminal, execute the following command:
node app.js
You should see a message indicating that the server is running:
Server is running on http://localhost:3000
Step 5: Testing Your Application
To test your application, open your web browser and navigate to http://your-server-ip:3000
. You should see “Hello, World!” displayed in your browser.
Step 6: Process Management with PM2 (Optional)
While you can run your application using the node
command in the terminal, it’s not recommended for production environments because it won’t automatically restart if the server crashes. For this reason, you may want to use a process manager like PM2.
- Install PM2 globally:
sudo npm install -g pm2
- Start your application using PM2:
pm2 start app.js
- To check the status:
pm2 status
- To set PM2 to restart on system boot:
pm2 startup
Follow the instructions PM2 provides after running this command to set up the startup script.
Conclusion
Congratulations! You have successfully installed Node.js on your CentOS server and created a simple application. You also learned how to use PM2 to manage your application processes more effectively.
Make sure to check back for more tutorials and tips on hosting and managing your applications at Greenhost.cloud! Happy coding!