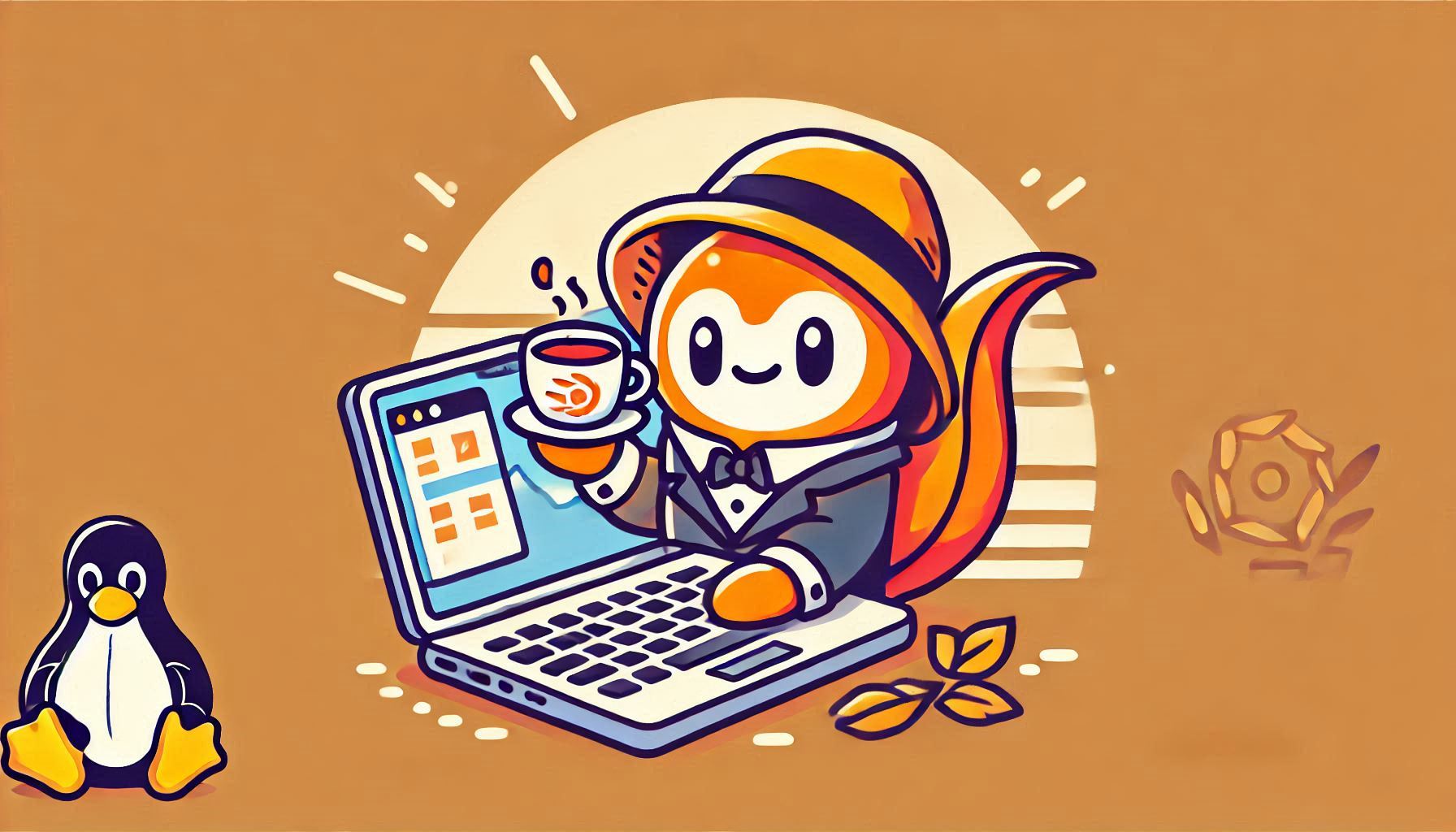
How To Use Memcached With Ruby on Rails on Ubuntu 24.04 or Newer
Welcome back to the Greenhost.cloud blog! Today, we delve into the world of caching in Ruby on Rails applications, specifically focusing on how to set up and use Memcached on Ubuntu 24.04 or newer. Memcached is a high-performance distributed memory caching system that can greatly enhance the performance of your web applications by reducing the load on your database and speeding up data retrieval.
What is Memcached?
Memcached is an in-memory key-value store that can significantly improve the speed of applications by caching frequently accessed data. It allows you to store data temporarily in RAM, which is much faster to access than querying a database. This is especially beneficial for read-heavy applications, where the same data is requested multiple times.
Why Use Memcached with Ruby on Rails?
Ruby on Rails is known for its ease of use and convention over configuration, but as your application grows, you may encounter performance bottlenecks, especially with database queries. Memcached can help alleviate these issues by:
- Reducing the number of database queries.
- Decreasing response times for end-users.
- Improving scalability as your application grows.
Prerequisites
Before we dive into the installation and setup process, ensure that you have the following:
- A server running Ubuntu 24.04 or newer.
- Ruby on Rails installed.
- Basic knowledge of Ruby on Rails and command line operations.
Step 1: Install Memcached
First, you need to install Memcached on your Ubuntu server. Open your terminal and run the following commands:
sudo apt update
sudo apt install memcached libmemcached-dev
Once the installation is complete, you can start and enable Memcached to run on boot:
sudo systemctl start memcached
sudo systemctl enable memcached
To check if Memcached is running, you can use:
sudo systemctl status memcached
You should see an output indicating that Memcached is active and running.
Step 2: Configure Memcached
The default Memcached configuration should work for most applications, but you can customize it by editing the configuration file. Open the file with your preferred text editor:
sudo nano /etc/memcached.conf
Here, you can adjust parameters such as the memory limit and port. The default settings are usually sufficient, but feel free to change the -m
option to allocate more memory if needed. After editing, save and exit the editor.
To apply changes, restart Memcached:
sudo systemctl restart memcached
Step 3: Add the Dalli Gem to Your Rails Application
Next, you will need to add the Dalli gem to your Rails application. Dalli is a modern, thread-safe client for Memcached.
Open your Gemfile located in the root directory of your Rails application and add the following line:
gem 'dalli'
Then, run the following command to install the gem:
bundle install
Step 4: Configure Rails to Use Memcached
Now that you have installed Memcached and the Dalli gem, you need to configure your Rails application to use Memcached for caching. Open config/environments/production.rb
(or development.rb
if you are working in a development environment) and add the following lines:
config.cache_store = :dalli_store, 'localhost:11211', { namespace: 'my_app', expires_in: 1.day, compress: true }
Make sure to replace 'my_app'
with your application’s name. The expires_in
option allows you to specify how long the cached data should be stored before it expires.
Step 5: Using Caching in Your Rails Application
With Memcached set up, you can now start caching data in your Rails application. Here are some examples of how to use caching:
Caching Action Results
You can cache the results of controller actions like this:
class ArticlesController < ApplicationController
def index
@articles = Rails.cache.fetch("articles/all", expires_in: 12.hours) do
Article.all.to_a
end
end
end
Caching Fragment Views
You can also cache specific parts of your views:
<% cache @article do %>
<h1><%= @article.title %></h1>
<p><%= @article.content %></p>
<% end %>
Clearing Cache
To clear the cache, you can use:
Rails.cache.delete("articles/all")
Conclusion
Congratulations! You have successfully set up Memcached with Ruby on Rails on your Ubuntu 24.04 or newer server. By implementing Memcached, you can enhance the performance of your application, providing a smoother experience for your users. Remember to monitor your cache usage and adjust your settings as necessary to optimize performance.