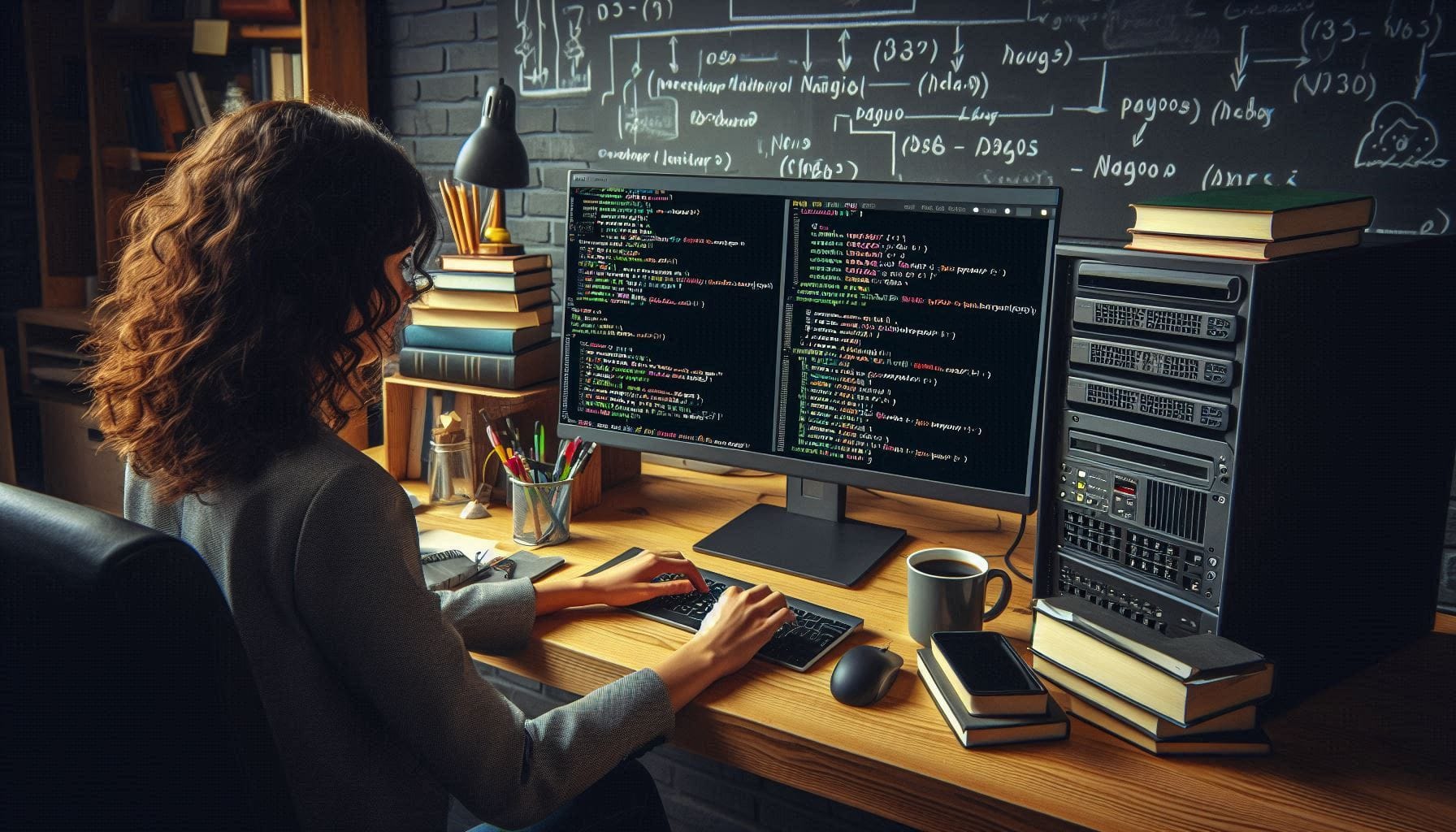
How To Create Nagios Plugins With Python On Ubuntu
Monitoring the performance and availability of servers and services is crucial for any organization. Nagios, a powerful monitoring tool, helps in this aspect by sending alerts when issues arise. While Nagios comes with built-in plugins to check various services, creating custom plugins can tailor the monitoring to specific needs. In this post, we will guide you through creating Nagios plugins using Python on an Ubuntu system.
What are Nagios Plugins?
Nagios plugins are executable scripts or binaries that return specific output to Nagios, which then decides whether to alert you based on the results. A standard Nagios plugin must return a single line of output that indicates its health status. The output typically follows three states:
- OK (0): The service is running as expected.
- WARNING (1): The service is experiencing mild issues.
- CRITICAL (2): The service is down or malfunctioning.
- UNKNOWN (3): The plugin cannot determine the state.
Prerequisites
Before diving into creating a Nagios plugin, ensure you have the following:
- An Ubuntu server with Nagios installed. If Nagios is not yet installed, follow these instructions:
sudo apt update
sudo apt install nagios3
- Python3 installed (usually pre-installed in Ubuntu environments):
python3 --version
- A basic understanding of Python programming.
Step 1: Setting Up the Plugin Directory
By default, Nagios looks for plugins in the /usr/lib/nagios/plugins/
directory. It is recommended to create your plugins here:
sudo mkdir -p /usr/lib/nagios/plugins/custom
sudo chown $USER:$USER /usr/lib/nagios/plugins/custom
Step 2: Creating the Python Plugin
Let’s create a simple Nagios plugin that checks the disk usage of the root filesystem and alerts if it exceeds a specified threshold.
- Open your favorite text editor and create a Python script:
nano /usr/lib/nagios/plugins/custom/check_disk_usage.py
- Add the following Python code to the file:
#!/usr/bin/env python3
import os
import sys
import shutil
# Define the threshold percentage
THRESHOLD = 80
# Get the disk usage statistics
total, used, free = shutil.disk_usage("/")
# Calculate the percentage of used space
percent_used = (used / total) * 100
# Generate output based on the used space
if percent_used > THRESHOLD:
print(f"CRITICAL - Disk usage is at {percent_used:.2f}%")
sys.exit(2)
elif percent_used > THRESHOLD - 20:
print(f"WARNING - Disk usage is at {percent_used:.2f}%")
sys.exit(1)
else:
print(f"OK - Disk usage is at {percent_used:.2f}%")
sys.exit(0)
- Save the file and exit the editor.
Step 3: Making the Script Executable
After creating the script, you need to make it executable:
sudo chmod +x /usr/lib/nagios/plugins/custom/check_disk_usage.py
Step 4: Configuring Nagios to Use the Plugin
Now that we have our plugin, we need to configure Nagios to use it:
- Open the Nagios configuration file for hosts or services. For example, if you want to check the disk usage of a specific host:
sudo nano /etc/nagios3/conf.d/localhost_nagios2.cfg
- Add the following definition to check disk usage:
define command {
command_name check_disk_usage
command_line /usr/lib/nagios/plugins/custom/check_disk_usage.py
}
define service {
use generic-service
host_name localhost
service_description Disk Usage
check_command check_disk_usage
}
- Save and close the configuration file.
Step 5: Verifying the Configuration and Restarting Nagios
To ensure that there are no errors in the configuration, run:
sudo nagios3 -v /etc/nagios3/nagios.cfg
If everything checks out, restart the Nagios service:
sudo systemctl restart nagios3
Step 6: Testing the Plugin
You can manually test your plugin by running it directly from the terminal:
/usr/lib/nagios/plugins/custom/check_disk_usage.py
You should see output similar to:
OK - Disk usage is at 45.50%
WARNING - Disk usage is at 78.90%
CRITICAL - Disk usage is at 85.00%
Conclusion
Creating Nagios plugins using Python on Ubuntu is a straightforward process that enhances your monitoring capabilities. By tailoring plugins to your specific needs, you can ensure that you’re always informed about the performance and health of your critical services.
If you enjoyed this tutorial, check out our other posts on monitoring tools and cloud solutions at Greenhost.cloud. Happy Monitoring!