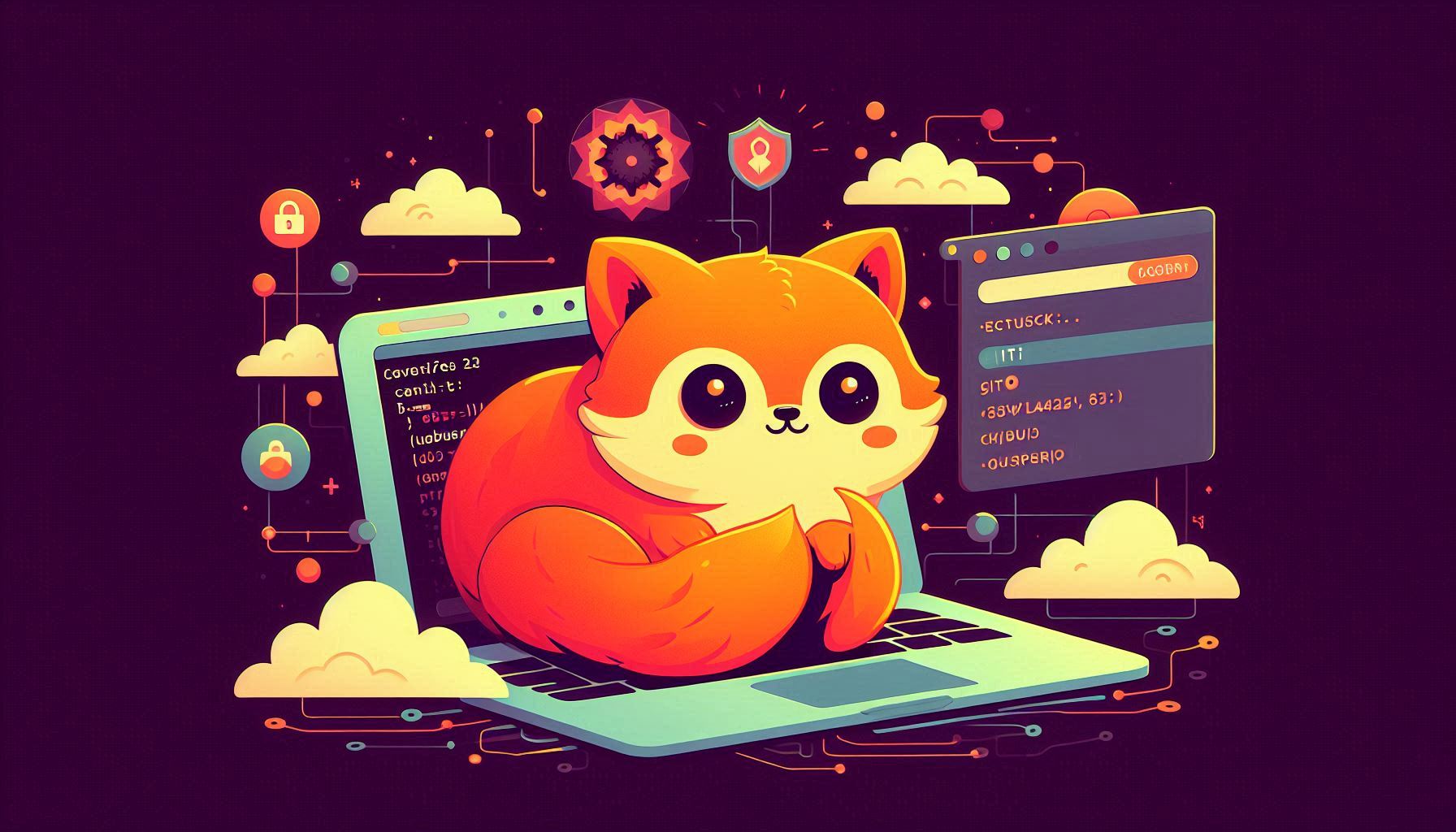
Welcome back to the Greenhost.cloud blog! Today, we’re diving into a practical guide on how to install Express, the popular Node.js framework, and set up Socket.io on Ubuntu 24.04 or newer. Whether you’re building a real-time chat application, a collaborative tool, or just want to enhance your web project with WebSocket capabilities, this guide will help you get started. Let’s jump in!
Prerequisites
Before we begin, ensure you have the following:
- Ubuntu 24.04 or newer installed and running.
- Node.js and npm (Node Package Manager) installed. If you don’t have them installed yet, follow these steps.
Step 1: Install Node.js and npm
- Update your package list:
sudo apt update
- Install Node.js. The following command will install the latest version of Node.js along with npm:
sudo apt install nodejs npm -y
- Verify the installation:
node -v
npm -v
You should see the versions of Node.js and npm displayed in your terminal.
Step 2: Set Up Your Project Directory
Let’s create a new directory for your project and navigate into it.
mkdir my-express-app
cd my-express-app
Step 3: Initialize a New Node.js Project
Use npm to initialize a new Node.js project. This will create a package.json
file where your project’s dependencies will be listed.
npm init -y
Step 4: Install Express
Now, let’s install Express, which is a web application framework for Node.js.
npm install express
Step 5: Create a Basic Express Server
Create a new file named server.js
in your project directory:
touch server.js
Open server.js
in your preferred text editor and add the following code:
const express = require('express');
const app = express();
const PORT = 3000;
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Step 6: Run Your Express Server
Now, you can start your Express server:
node server.js
Open your web browser and navigate to http://localhost:3000
. You should see “Hello, Express!” displayed on your screen.
Step 7: Install Socket.io
To add real-time capabilities to your application, let’s install Socket.io.
npm install socket.io
Step 8: Set Up Socket.io
Now that Socket.io is installed, let’s modify our server.js
file to include Socket.io functionality.
Update your server.js
to look like this:
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
const PORT = 3000;
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('disconnect', () => {
console.log('User disconnected');
});
});
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Step 9: Create an HTML File
Create a new file named index.html
in your project directory:
touch index.html
Open index.html
and add the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Socket.io Example</title>
<script src="/socket.io/socket.io.js"></script>
<script>
var socket = io();
</script>
</head>
<body>
<h1>Welcome to the Socket.io Example</h1>
</body>
</html>
Step 10: Test Socket.io
Restart your server if it’s running:
node server.js
Visit http://localhost:3000
in your browser. Open the console (right-click and select “Inspect” > “Console”) to see the log message indicating that a user has connected.
Conclusion
Congratulations! You have successfully installed Express, set up a basic server, and integrated Socket.io on Ubuntu 24.04 or newer. You can now start building real-time applications that can communicate seamlessly with clients.
At Greenhost.cloud, we believe in empowering developers with the right tools and resources. Feel free to explore more about Express and Socket.io, and don’t hesitate to reach out if you have any questions!
Happy coding!